Creating a 3d printable lithophane in OpenSCAD is relatively easy. But in this post we use a trick to create a curved lithophane.
Tag: FLOSS
I found an example on Thingiverse, a rubber band powered boat with two peddle wheels, but it has two problems. First of all the author only provides .stl files and second the design is a bit flawed. I therefore decided to design the boat from scratch with the 3d CAD program OpenSCAD. With OpenSCAD I’m […]
Moving to Free and Open Source on the PC can be a very daunting task for someone that is used to a proprietary system. This is how I did it.
Why create your own music library and why use the free and open source MusicBrainz Picard for this.
Parametric design of a simple hook in, the free and open source, OpenSCAD. Use with the Customizer in OpenSCAD
In this Solvespace new series of video tutorials I’ll demonstrate how to create an involute gear, adjust an existing involute gear and demonstrate how one involute gear drives another.
OpenSCAD allows the user to create complex shapes with the polygon function for 2D and polyhedron for 3D. Polygon and polyhedron both accept a list of 2D and 3D coordinates (points) respectively as parameters. A functions can generate a list of points eliminating the need to manually created these lists. This property can be used […]
A few years ago I wrote about my IBM Thinkpad happily running Puppy Linux. The laptop is still running fine but now with AntiX.
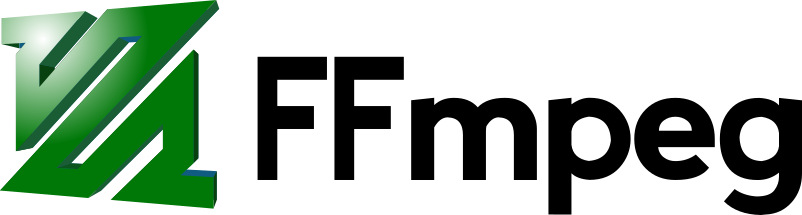
Learn how to create an animated GIF that’s small enough to upload but with a decent quality.
With G+ closing in a couple of months community owners are searching for alternatives. I provide a number of FLOSS options.